Asynchronous search results with JQuery, Solr, Json and Ajax
Update: Aug 5th 2012 A live demo of asynchronous search results with JQuery, Solr, Json and Ajax can now be found here
There are many reasons to load search results asynchronously- most of the big searchy dotcoms are going in this direction with Google, Facebook and Twitter all relying heavily on ajax to render their feed-based pages.
Considering how mainstream it has become, information on the nuts and bolts of the technique remain thin on the ground. So here is a really quick overview of what you need to get started.
For the purposes of this post I will assume that you have an working installation of Solr in a more or less standard configuration. Getting Solr up and running is no mean feat in itself, but mercifully there are a number of fairly decent guides squirreled away on the internet. You could of course use any search motor that returns search results in a Json format, in fact even if they dont, Comperio Front can now be used to provide a JSON query service on any (collection of) search motor(s).
So lets assume that a query for “Ford” returns Json in the following format from our very small index of cars. Note that the Solr URL for this feed would look something like this: http://localhost:8080/solr/select/?q=ford&wt=json&json.wrf=?&indent=true where wt specifies json and json.wrf specifies the callback
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
({ "responseHeader":{ "status":0, "QTime":1, "params":{ "json.wrf":"?", "indent":"true", "q":"ford", "wt":"json"}}, "response":{"numFound":3,"start":0,"docs":[ { "id":"147", "name":"Ford Capri", "description":"pink 1978 model- great condition", "owner":"576463865", "store":"59.7440738,10.204456400000026", "tags":[ "Ford, Capri, Pink, 1978"]}, { "id":"148", "name":"Ford Orion", "description":"Blue, Good runner", "owner":"576463865", "store":"59.9138688,10.752245399999993", "tags":[ "blue, orion, 1.6"]}, { "id":"149", "name":"Ford Anglia", "description":"As seen in Harry Potter, Can be flown", "owner":"576463865", "store":"57.2366283,-2.2650615999999673", "tags":[ "Harry Potter, Anglia, Flying car"]} ] } }) |
We can now parse this feed by using the .getJSON function in the excellent jQuery library. The javascript and associated HTML would be more or less like so:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<div id="rs"></div> <script type='text/javascript' src='//ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.js'></script> <script type='text/javascript'> $.getJSON("http://localhost:8080/solr/select/?q=ford&wt=json&json.wrf=?&indent=true", function(result){ var Parent = document.getElementById('rs'); for (var i = 0; i < result.response.docs.length; i++) { var thisResult = "<b>" + result.response.docs[i].name + "</b><br>" + result.response.docs[i].description + ", " + result.response.docs[i].tags + "<br>"; var NewDiv = document.createElement("DIV"); NewDiv.innerHTML = thisResult; Parent.appendChild(NewDiv); } }); </script> |
And thats it :-) You will notice that when the HTML above is loaded in a browser, that the search results are returned independently of the webpage.
Next Steps: Use JavaScript templating to render results from Solr
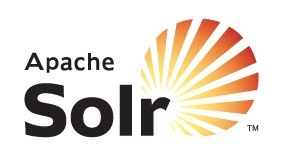
None more AJAXy than Google these days, with their Instant Search, although Yahoo! Search Direct is a good number 2.
http://www.youtube.com/watch?v=NvoNFP-v7v8
The SERP with a page load (perceived as a noticable flicker) may be an essential part of the users expectation, and AJAX may actually violate that expectation if done without care. At least some form of visual feedback, usually a spinner, must be provided to help the user direct his/her attention to the update.
I’d love to see your example done with the Templates plugin for jQuery. That should clean up your code nicely :-)
http://api.jquery.com/category/plugins/templates/
I must admit that I had to google SERP ;-)
The example above would give you a page analogous to facebook’s “news feed” (home page)- so the frame loads fairly instantaneously and the results populate it afterwards.